Table of Contents
Node.js thrives on asynchronous, event-driven development. Unlike the traditional blocking approach, it handles multiple connections concurrently on a single thread thanks to its non-blocking I/O model. Understanding the the Node.js event loop is crucial for building responsive and performant applications even under heavy loads.
In this blog post, we'll delve into the intricacies of the Node.js event loop, its components and lifecycle. Whether you're a Node.js seasoned developer or a curious newcomer, this guide will provide you with the knowledge you need to harness the full power of Node.js.
>> You may be interested in:
- Top 9 Best Node.js Frameworks For Web App Development
- Top 15 Node.js Projects for Beginners and Professionals
What is the Event Loop?
The event loop is the beating heart of every Node.js application, orchestrating the flow of asynchronous operations and ensuring that your code runs smoothly without blocking execution. At its core, the event loop is a continuous process that monitors the execution stack and manages the flow of tasks in a non-blocking manner.
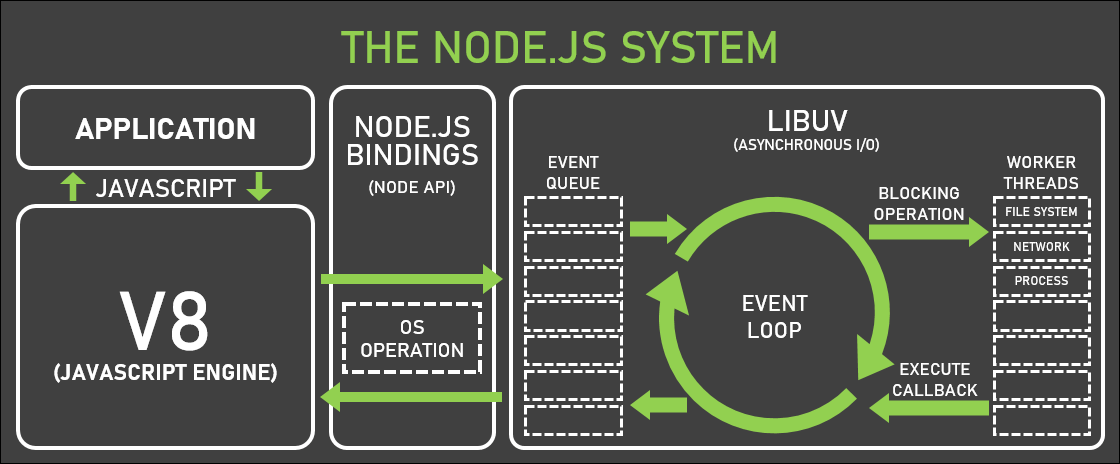
In traditional synchronous programming models, when a function is called, the execution is blocked until that function returns. This means that if a function takes a long time to execute, it can hold up the entire program. However, in Node.js, things work differently.
The event loop in Node.js allows the execution of multiple tasks concurrently on a single thread. Instead of waiting for a task to complete before moving on to the next one, Node.js delegates tasks to the operating system and continues executing other code. When a task completes, its corresponding callback function is placed in the event queue to be processed later by the event loop.
How the Event Loop Works?
Node.js is particularly well-suited for I/O-bound and event-driven applications, such as web servers or real-time communication systems, where many operations can be performed concurrently without CPU-intensive computations.
The event loop's ability to delegate I/O operations and schedule callback functions enables Node.js to make efficient use of system resources, maximizing throughput and minimizing latency. This asynchronous, non-blocking architecture is one of the key reasons why Node.js is widely used for building scalable and high-performance applications.
Comparison with Traditional Synchronous Programming Models
In traditional synchronous programming, the flow of execution is straightforward and predictable. Each line of code is executed sequentially, and functions are called and completed in order.
However, in asynchronous programming with Node.js, the event loop introduces a new paradigm. Tasks are executed asynchronously, and the event loop manages the flow of these tasks, ensuring that the program remains responsive even while handling multiple operations concurrently. This non-blocking nature allows Node.js to handle high levels of concurrency efficiently.
Key Components of the Event Loop
The event loop in Node.js consists of several key components that work together to manage the flow of asynchronous operations and ensure efficient execution. Let's explore these components in detail:
Execution Stack
The execution stack, also known as the call stack, is a data structure that tracks the execution context of synchronous code in Node.js. When a function is called, a new frame is pushed onto the stack, representing the execution context of that function. As functions complete, their frames are popped off the stack.
The event loop begins by checking the execution stack. If the stack is empty, it moves on to the next phase of the event loop, otherwise, it waits for the stack to become empty before proceeding.
Event Queue
The event queue, sometimes referred to as the message queue, is where callback functions from asynchronous operations are queued for execution. When an asynchronous operation completes, such as reading from a file or making an HTTP request, its corresponding callback function is placed in the event queue.
The event loop continuously checks the event queue for any tasks waiting to be processed. If the stack is empty, it retrieves tasks from the event queue and pushes them onto the execution stack for execution.
Timers and I/O Events
In addition to managing callback functions from asynchronous operations, the event loop also handles timers and I/O events. Timers, created using functions like setTimeout() and setInterval(), schedule tasks to be executed after a specified delay. When a timer expires, its corresponding callback function is added to the event queue for processing.
Similarly, I/O operations such as reading from a file or making a network request are handled asynchronously by Node.js. When an I/O operation completes, its callback function is queued in the event queue for execution by the event loop.
Understanding Asynchronous Operations
Asynchronous operations lie at the core of Node.js's ability to handle concurrent tasks efficiently. Let's delve into two key aspects of asynchronous programming in Node.js:
Non-blocking I/O
Node.js excels at handling I/O operations in a non-blocking manner. Traditionally, in synchronous programming, I/O operations like reading from a file or making a network request would block the execution of the program until the operation is complete. This can lead to inefficiencies, especially in scenarios where multiple I/O operations are involved.
However, in Node.js, I/O operations are delegated to the underlying system, allowing the program to continue executing other tasks while waiting for the I/O operation to complete. This non-blocking nature ensures that the program remains responsive and can handle multiple operations concurrently.
For example, when a Node.js application needs to read data from a file, instead of waiting for the file read operation to complete, it initiates the operation and provides a callback function to be executed once the operation is finished. In the meantime, the program can continue executing other tasks, maximizing throughput and efficiency.
Callbacks
Callback functions play a central role in asynchronous programming in Node.js. A callback function is a function that is passed as an argument to another function and is executed once the asynchronous operation associated with that function is complete.
In Node.js, callback functions are commonly used to handle the results of asynchronous operations such as I/O operations, timer events, or network requests. When an asynchronous operation completes, it invokes the callback function, passing any relevant data as arguments.
For example, when reading data from a file asynchronously in Node.js, you would typically provide a callback function that receives the data read from the file as an argument. This allows you to process the data once it's available, without blocking the execution of the program.
Example Code Snippets
// Example of non-blocking I/O (reading from a file)
const fs = require('fs');
// Asynchronously read data from a file
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log('File contents:', data);
});
console.log('Reading file...');
// Output:
// Reading file...
// File contents: Hello, world!
Common Misconceptions of Node.js Event Loop
- Blocking the Event Loop: One common misconception is that blocking operations such as CPU-intensive computations will halt the event loop. While Node.js excels at handling I/O-bound tasks asynchronously, blocking the event loop with long-running operations can degrade performance and responsiveness.
- Callback Hell: In complex Node.js applications, nested callbacks can lead to a phenomenon known as "callback hell," making the code difficult to read and maintain. This can occur when handling multiple asynchronous operations sequentially. Fortunately, modern JavaScript features like Promises and async/await offer solutions to mitigate callback hell.
- Overusing Synchronous Operations: Node.js provides synchronous versions of many functions for convenience, but overusing synchronous operations can lead to decreased performance. It's essential to favor asynchronous operations, especially for I/O-bound tasks, to ensure that the event loop remains responsive.
Pitfalls to Avoid When Working with Asynchronous Code
- Neglecting Error Handling: Failing to handle errors properly in asynchronous code can lead to unpredictable behavior and vulnerabilities. Always ensure that error objects are properly handled and propagated to avoid silent failures.
- Leaking Resources: Asynchronous operations like opening files or creating network connections allocate system resources. Failing to release these resources properly can lead to resource leaks and eventual exhaustion. Utilize patterns like resource pooling and proper cleanup to mitigate this risk.
- Not Understanding Execution Context: In Node.js, asynchronous code can introduce subtle issues related to variable scope and execution context. Understanding concepts like closures and the event loop's execution model is crucial for writing robust asynchronous code.
>> Read more about Node.js coding:
Conclusion
We've journeyed through the Node.js event loop, uncovering its role in managing asynchronous operations efficiently. Remember:
- The event loop orchestrates tasks, ensuring non-blocking execution.
- Components like the execution stack and event queue handle tasks seamlessly.
- Asynchronous operations and callbacks empower responsive code.
Avoid common pitfalls, deepen your understanding, and share your experiences. Keep exploring, keep coding, and let's continue the discussion!
>>> Follow and Contact Relia Software for more information!
- coding
- development